How to get the expiration date of multiple domains using Python?
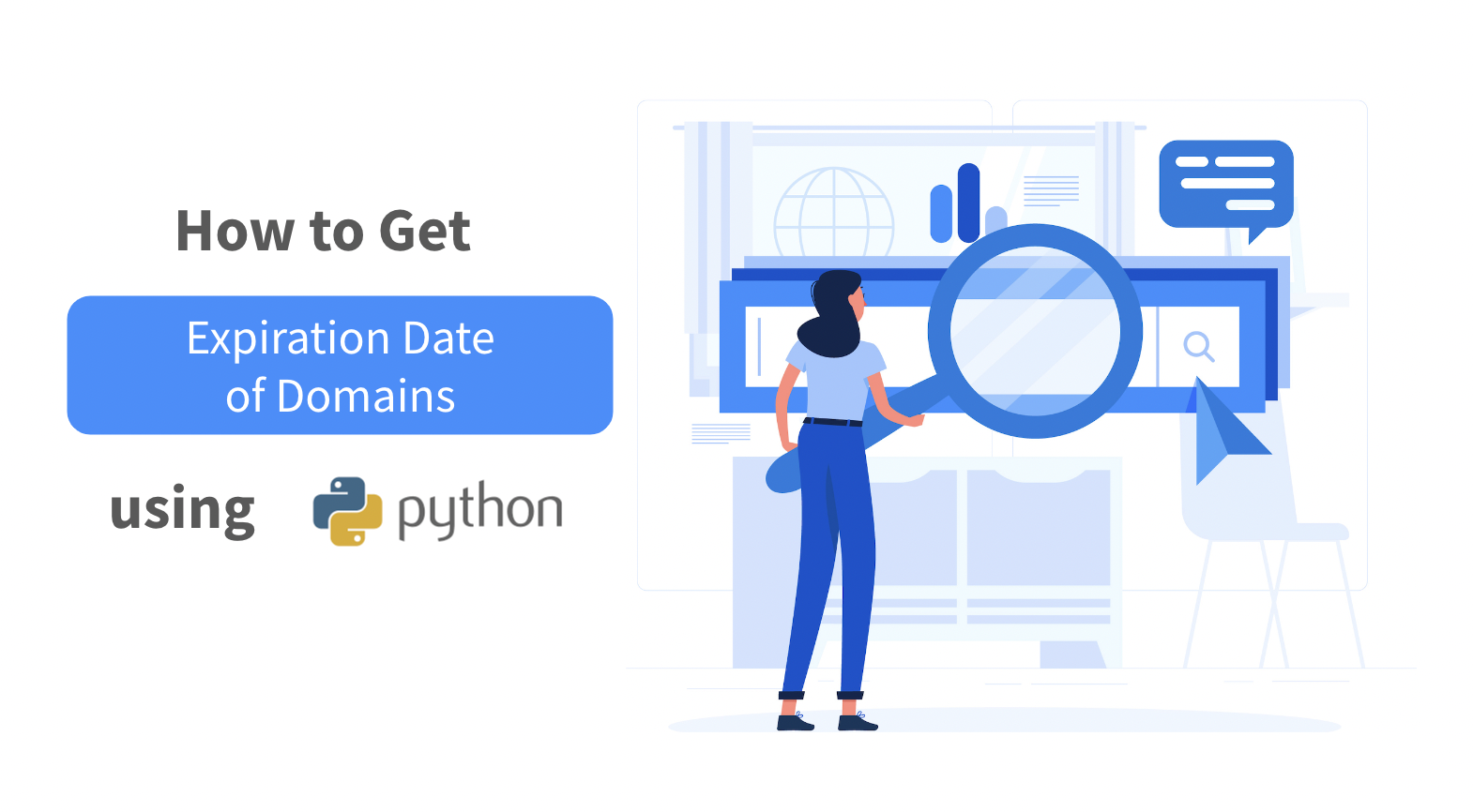
You may have been tasked with retrieving the expiration date of multiple domains. Companies often have multiple domains, requiring careful monitoring of their expiration dates to prevent them from expiring. Additionally, you might be an agency offering renewal services to your clients. Regardless of the use case, if you're a Python programmer, there's a simple way to fetch the expiration dates of all the domains you need. It's straightforward; you just need to integrate with the Klazify API using Python and make the appropriate request. Here's how to do it step by step:
1. First of all, go to http://Klazify.com.
2. Click on the "GET API KEY" button and complete the SIGN UP process.
3. After signing up, you'll need to enter your card details to subscribe to a plan. Don't worry; you have a 7-DAY FREE TRIAL. This means you can try the API for 7 days without any charges! This gives you time to integrate and test the API to see if it meets your needs.
4. Once subscribed to a plan, you can access your API Access Key (you can find it in the Dashboard menu option). This is the key that allows you to make queries to the API.
5. Now you can start the integration. Scroll down to the "Examples" section in the Dashboard.
6. Select the "Domain Expiration API" endpoint. (The API has many more endpoints that we will cover in other articles!).
7. After selecting the endpoint, you'll see the Code Snippet for integrating the API in PHP, Python, JavaScript jQuery AJAX, etc. We'll focus on Python for this article.
Python
import requests
url = "https://www.klazify.com/api/domain_expiration"
payload = "{\"url\":\"https://www.google.com\"}\n"
headers = {
\'Accept\': "application/json",
\'Content-Type\': "application/json",
\'Authorization\': "Bearer access_key",
\'cache-control\': "no-cache"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
8. Note that where it says: "'Authorization': "Bearer access_key", you should replace "access_key" with the API Key mentioned in step 4.
And that's it! You can now start using the API.
Below are some examples of the response the API will provide for the URLs http://cnn.com and http://google.com.
Example API Response (for cnn.com)
{
"domain": {
"domain_url": "http://cnn.com"
},
"success": true,
"domain_registration_data": {
"domain_age_date": "1993-09-22",
"domain_age_days_ago": 11069,
"domain_expiration_date": "2027-09-21",
"domain_expiration_days_left": 1347
}
}
Example API Response (for google.com)
{
"domain": {
"domain_url": "https://google.com"
},
"success": true,
"domain_registration_data": {
"domain_age_date": "1997-09-15",
"domain_age_days_ago": 9228,
"domain_expiration_date": "2028-09-13",
"domain_expiration_days_left": 2092
}
}
I hope this helps! If you have any questions, feel free to contact support via the chat at http://Klazify.com or send an email with your query to [email protected]!
Have a great integration!
Ready to use Klazify?
Try it now!Recent Posts
- URL Data Extraction APIs for Content Analysis
- URL Data Extraction API Automates Information Gathering
- URL Data Extraction APIs for Accurate Data Collection
- Website Data Enrichment API: Tips To Use It
- Website Data Enrichment API in Customer Segmentation
- Website Data Enrichment API for B2B Companies
- Website Data Enrichment API for Business Growth
- Website Data Enrichment API for Real Time Insights
- Website Data Enrichment API for 2025
- Website Data Enrichment API for Better Customer Insights